Categories: HTML5; Tagged with: HTML5 • Polymer; @ October 15th, 2015 22:26Dispatching Event:
<link rel="import" href="../bower_components/polymer/polymer.html" />
<link rel="import" href="../bower_components/iron-icons/iron-icons.html" >
<link rel="import" href="../bower_components/paper-button/paper-button.html" />
<dom-module id="item-detail">
<template>
<div id="container">
<h3>{{item_id}}</h3>
</div>
<paper-button on-tap="onAddToCart"><iron-icon icon="icons:shopping-cart"></iron-icon>AddToCart</paper-button>
</template>
<script>
Polymer({
is: 'item-detail',
properties: {
item_id: {
type:Number,
value:-1
}
},
onAddToCart: function(e) {
console.log('adding...');
this.fire('eventAddToCart', {item_id: this.item_id});
}
});
</script>
</dom-module>
Event Listener:
<link rel="import" href="bower_components/polymer/polymer.html" />
<link rel="import" href="element/item-detail.html">
<dom-module id="main-app">
<template>
<item-detail id="item_detail" item_id="{{current_detail_id}}"></
</template>
<script>
Polymer({
is: 'main-app',
listeners: {
'item_detail.eventAddToCart': 'onAddToCart'
},
onAddToCart: function(e) {
console.log('Event received' + e.detail.item_id);
// this.addToCart(e.detail.item_id);
}
});
</script>
</dom-module>
Categories: Android • Development Notes • HTML5; Tagged with: Android • Apache Cordova • HTML5 • iOS; @ July 21st, 2015 23:14Requirement: Build iOS/Android app using existing Polymer project.
Solution:
There’re lots of tools/platforms to build an app from HTML5 project, such as Trigger.io, Intel XDK, PhoneGap, Apache Cordova. I choosed Cordova to build my first html app;
- Install Apache Cordova by npm:
$ sudo npm install -g cordova
- Create a Cordova project using cordova CLI;
$ cordova create hello com.liguoliang.app HelloWorld
- Navigate to the project folder, add platform:
$ cordova platform add ios/android
- Build project:
$ cordova build
- Emulate the app:
$ cordova emulate ios/andorid
Note: It is required to install Xcode or Android SDK/Tools for simulating. you may follow the Cordova CLI message to installed the required sdk/tool.
> Apache Cordova CLI Document
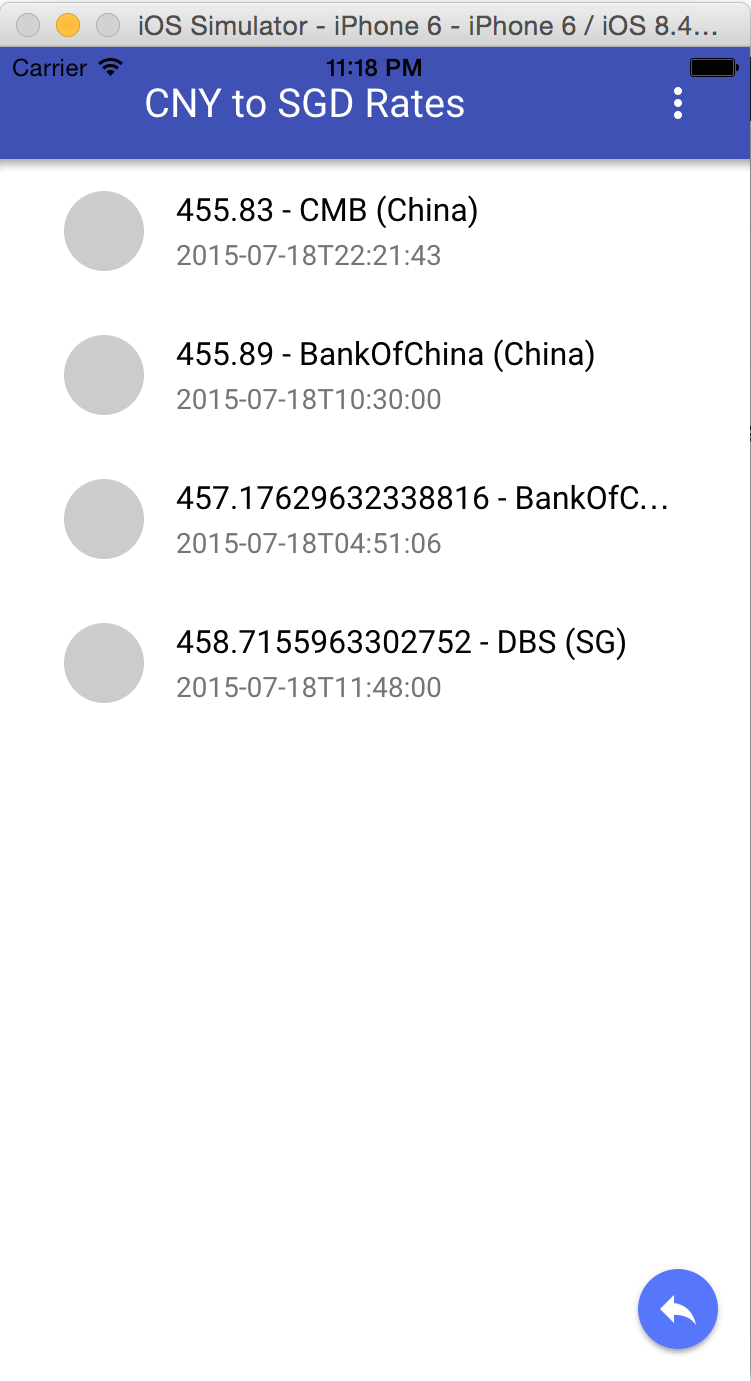
Categories: Development Notes • HTML5; Tagged with: HMTL5 • HTML • Polymer • WebComponent; @ July 19th, 2015 23:10
Requirement: creating a component to display user info
Step 1: Create a web component using dom-module:
user-info.html:
<link rel="import" href="../bower_components/polymer/polymer.html" />
<dom-module id="user-info">
<template>
<div id="container">
Hi, <span>{{user_name}}</span>!
</div>
</template>
<style>
#container {
font-size: 11px;
color: gray;
}
</style>
<script>
Polymer({
is: 'user-info',
properties: {
user_name: {
type:String,
value:"Mr.default"
}
}
});
</script>
</dom-module>
Step 2: Use the newly created component:
<link rel="import" href="components/user-info.html" />
<template is="dom-bind">
<user-info user_name="Li"></user-info>
</template>
Categories: JavaScript; Tagged with: JS; @ November 20th, 2014 23:35Array.Map:
Array.prototype.map = function(projectionFunction) {
var results = [];
this.forEach(function(itemInArray) {
// ------------ INSERT CODE HERE! ----------------------------
// Apply the projectionFunction to each item in the array and add
// each result to the results array.
// Note: you can add items to an array with the push() method.
// ------------ INSERT CODE HERE! ----------------------------
results.push(projectionFunction(itemInArray));
});
return results;
};
// JSON.stringify([1,2,3].map(function(x) { return x + 1; })) === '[2,3,4]'
Array.filter
Array.prototype.filter = function(predicateFunction) {
var results = [];
this.forEach(function(itemInArray) {
// ------------ INSERT CODE HERE! ----------------------------
// Apply the predicateFunction to each item in the array. If the
// result is truthy, add each result to the results array.
// Note: remember you can add items to the array using the array's
// push() method.
// ------------ INSERT CODE HERE! ----------------------------
if(predicateFunction(itemInArray)) {
results.push(itemInArray);
}
});
return results;
};
// JSON.stringify([1,2,3].filter(function(x) { return x > 2})) === "[3]"
Chaining call
function() {
var newReleases = [
{
"id": 70111470,
"title": "Die Hard",
"boxart": "http://cdn-0.nflximg.com/images/2891/DieHard.jpg",
"uri": "http://api.netflix.com/catalog/titles/movies/70111470",
"rating": 4.0,
"bookmark": []
},
{
"id": 654356453,
"title": "Bad Boys",
"boxart": "http://cdn-0.nflximg.com/images/2891/BadBoys.jpg",
"uri": "http://api.netflix.com/catalog/titles/movies/70111470",
"rating": 5.0,
"bookmark": [{ id:432534, time:65876586 }]
},
{
"id": 65432445,
"title": "The Chamber",
"boxart": "http://cdn-0.nflximg.com/images/2891/TheChamber.jpg",
"uri": "http://api.netflix.com/catalog/titles/movies/70111470",
"rating": 4.0,
"bookmark": []
},
{
"id": 675465,
"title": "Fracture",
"boxart": "http://cdn-0.nflximg.com/images/2891/Fracture.jpg",
"uri": "http://api.netflix.com/catalog/titles/movies/70111470",
"rating": 5.0,
"bookmark": [{ id:432534, time:65876586 }]
}
];
// ------------ INSERT CODE HERE! -----------------------------------
// Chain the filter and map functions to select the id of all videos
// with a rating of 5.0.
return newReleases.
filter(function(video) {
return video.rating === 5.0;
}).
map(function(video) {
return video.id;
});
// ------------ INSERT CODE HERE! -----------------------------------
}
Categories: JavaScript; Tagged with: JS; @ November 13th, 2014 22:57 // define a flight
var flight = {
airline: "KLM",
number: 835,
departure: {
city: "SG",
IATA: "SIN"
},
scheduleDays: [1, 2, 5]
};
console.log("Flight: ", flight);
// add new attribute
flight.code="KLM835";
console.log("Added code: ", flight);
// retrieve attribute
console.log("Retrieve departure city:", flight.departure.city);
// delete attribute
console.log("Flight code: ", flight.code);
delete flight.code;
console.log("Deleted flight code: ", flight.code);
// for...in
for(day in flight.scheduleDays) {
console.log("for...in: schedule day: ", day);
}
// for loop
for(i = 0; i < flight.scheduleDays.length; i++ ) {
console.log("Schedule day:", flight.scheduleDays[i], " @ index: " , i);
}
// define new function
var printFlight = function(flight) {
return "Flight print: " + flight.airline + " - " + flight.number;
};
// initialize new flight via prototype;
var flight2 = Object.create(flight);
console.log("Flight2 created based on Flight: " + printFlight(flight2));
// add new attribute to the new flight
flight2.code = "KML836";
console.log("Flight2.code: ", flight2.code);
console.log("Flight.code: ", flight.code);
// update new flight
flight2.airline = "SIA";
console.log("Flight.airline: ", flight.airline);
console.log("Flight2 airline", flight2.airline);
// update the prototype;
flight.airline = "BA";
console.log("Flight.airline: ", flight.airline);
console.log("Flight2 airline", flight2.airline);
// type of
console.log(typeof flight);
console.log(typeof flight2);
console.log(typeof flight.scheduleDays[0]);
output:
Flight:
Object {airline: "KLM", number: 835, departure: Object, scheduleDays: Array[3]}
Added code:
Object {airline: "KLM", number: 835, departure: Object, scheduleDays: Array[3], code: "KLM835"}
Retrieve departure city: SG
Flight code: KLM835
Deleted flight code: undefined
for...in: schedule day: 0
for...in: schedule day: 1
for...in: schedule day: 2
Schedule day: 1 @ index: 0
Schedule day: 2 @ index: 1
Schedule day: 5 @ index: 2
Flight2 created based on Flight: Flight print: KLM - 835
Flight2.code: KML836
Flight.code: undefined
flight.airline: KLM
Flight2 airline SIA
Flight.airline: BA
Flight2 airline SIA
object
object
number
Older Posts